- class
Manager
¶
A Manager
is the interface through which database query operations areprovided to Django models. At least one Manager
exists for every model ina Django application.
The way Manager
classes work is documented in Making queries;this document specifically touches on model options that customize Manager
behavior.
URL Manager Pro 5.6. The popular and award-winning bookmark manager now available in 64-bit version for the latest macOS with support for Retina displays, Auto Save and Versions.URL Manager Pro allows you to manage your bookmarks independently from a web browser, yet use your bookmarks. URL Manager Pro is a bookmark manager for Safari, Explorer, OmniWeb, Mozilla, Netscape, iCab, Camino, and Epic browsers, making. It easier to manage bookmarks, email addresses and news groups.
Manager names¶
By default, Django adds a Manager
with the name objects
to every Djangomodel class. However, if you want to use objects
as a field name, or if youwant to use a name other than objects
for the Manager
, you can renameit on a per-model basis. To rename the Manager
for a given class, define aclass attribute of type models.Manager()
on that model. For example:
Using this example model, Person.objects
will generate anAttributeError
exception, but Person.people.all()
will provide a listof all Person
objects.
Custom managers¶
You can use a custom Manager
in a particular model by extending the baseManager
class and instantiating your custom Manager
in your model.
There are two reasons you might want to customize a Manager
: to add extraManager
methods, and/or to modify the initial QuerySet
the Manager
returns.
Adding extra manager methods¶
Adding extra Manager
methods is the preferred way to add “table-level”functionality to your models. (For “row-level” functionality – i.e., functionsthat act on a single instance of a model object – use Model methods, not custom Manager
methods.)
For example, this custom Manager
adds a method with_counts()
:
With this example, you’d use OpinionPoll.objects.with_counts()
to get aQuerySet
of OpinionPoll
objects with the extra num_responses
attribute attached.
A custom Manager
method can return anything you want. It doesn’t have toreturn a QuerySet
.
Another thing to note is that Manager
methods can access self.model
toget the model class to which they’re attached.
Modifying a manager’s initial QuerySet
¶

A Manager
’s base QuerySet
returns all objects in the system. Forexample, using this model:
…the statement Book.objects.all()
will return all books in the database.
You can override a Manager
’s base QuerySet
by overriding theManager.get_queryset()
method. get_queryset()
should return aQuerySet
with the properties you require.
For example, the following model has twoManager
s – one that returnsall objects, and one that returns only the books by Roald Dahl:
With this sample model, Book.objects.all()
will return all books in thedatabase, but Book.dahl_objects.all()
will only return the ones written byRoald Dahl.
Because get_queryset()
returns a QuerySet
object, you can usefilter()
, exclude()
and all the other QuerySet
methods on it. Sothese statements are all legal:
This example also pointed out another interesting technique: using multiplemanagers on the same model. You can attach as many Manager()
instances toa model as you’d like. This is a non-repetitive way to define common “filters”for your models.

For example:
This example allows you to request Person.authors.all()
, Person.editors.all()
,and Person.people.all()
, yielding predictable results.
Default managers¶
Model.
_default_manager
¶
If you use custom Manager
objects, take note that the first Manager
Django encounters (in the order in which they’re defined in the model) has aspecial status. Django interprets the first Manager
defined in a class asthe “default” Manager
, and several parts of Django (includingdumpdata
) will use that Manager
exclusively for that model. As aresult, it’s a good idea to be careful in your choice of default manager inorder to avoid a situation where overriding get_queryset()
results in aninability to retrieve objects you’d like to work with.
You can specify a custom default manager using Meta.default_manager_name
.
If you’re writing some code that must handle an unknown model, for example, ina third-party app that implements a generic view, use this manager (or_base_manager
) rather than assuming the model has an objects
manager.
Base managers¶
Model.
_base_manager
¶
Using managers for related object access¶
By default, Django uses an instance of the Model._base_manager
managerclass when accessing related objects (i.e. choice.question
), not the_default_manager
on the related object. This is because Django needs to beable to retrieve the related object, even if it would otherwise be filtered out(and hence be inaccessible) by the default manager.
If the normal base manager class (django.db.models.Manager
) isn’tappropriate for your circumstances, you can tell Django which class to use bysetting Meta.base_manager_name
.
Base managers aren’t used when querying on related models, or whenaccessing a one-to-many or many-to-many relationship. For example, if the Question
modelfrom the tutorial had a deleted
field and a basemanager that filters out instances with deleted=True
, a queryset likeChoice.objects.filter(question__name__startswith='What')
would includechoices related to deleted questions.
Don’t filter away any results in this type of manager subclass¶
This manager is used to access objects that are related to from some othermodel. In those situations, Django has to be able to see all the objects forthe model it is fetching, so that anything which is referred to can beretrieved.
Therefore, you should not override get_queryset()
to filter out any rows.If you do so, Django will return incomplete results.
Calling custom QuerySet
methods from the manager¶
While most methods from the standard QuerySet
are accessible directly fromthe Manager
, this is only the case for the extra methods defined on acustom QuerySet
if you also implement them on the Manager
:
This example allows you to call both authors()
and editors()
directly fromthe manager Person.people
.
Creating a manager with QuerySet
methods¶
In lieu of the above approach which requires duplicating methods on both theQuerySet
and the Manager
, QuerySet.as_manager()
can be used to create an instanceof Manager
with a copy of a custom QuerySet
’s methods:
The Manager
instance created by QuerySet.as_manager()
will be virtuallyidentical to the PersonManager
from the previous example.
Not every QuerySet
method makes sense at the Manager
level; forinstance we intentionally prevent the QuerySet.delete()
method from being copied ontothe Manager
class.
Methods are copied according to the following rules:
- Public methods are copied by default.
- Private methods (starting with an underscore) are not copied by default.
- Methods with a
queryset_only
attribute set toFalse
are always copied. - Methods with a
queryset_only
attribute set toTrue
are never copied.
For example:
from_queryset()
¶
- classmethod
from_queryset
(queryset_class)¶
For advanced usage you might want both a custom Manager
and a customQuerySet
. You can do that by calling Manager.from_queryset()
whichreturns a subclass of your base Manager
with a copy of the customQuerySet
methods:
You may also store the generated class into a variable:
Custom managers and model inheritance¶
Here’s how Django handles custom managers and model inheritance:
Url Manager Pro 5 1 – Bookmark Manager Job
- Managers from base classes are always inherited by the child class,using Python’s normal name resolution order (names on the childclass override all others; then come names on the first parent class,and so on).
- If no managers are declared on a model and/or its parents, Djangoautomatically creates the
objects
manager. - The default manager on a class is either the one chosen with
Meta.default_manager_name
, or the first managerdeclared on the model, or the default manager of the first parent model.
These rules provide the necessary flexibility if you want to install acollection of custom managers on a group of models, via an abstract baseclass, but still customize the default manager. For example, suppose you havethis base class:
If you use this directly in a subclass, objects
will be the defaultmanager if you declare no managers in the base class:
If you want to inherit from AbstractBase
, but provide a different defaultmanager, you can provide the default manager on the child class:
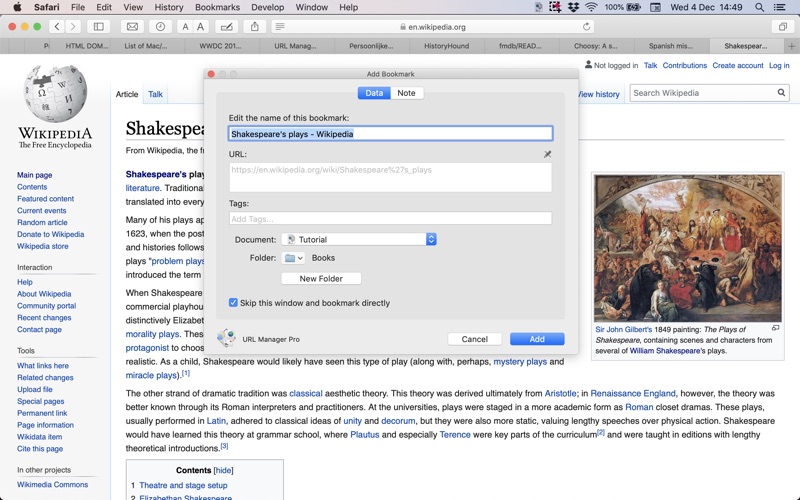
Here, default_manager
is the default. The objects
manager isstill available, since it’s inherited, but isn’t used as the default.
Finally for this example, suppose you want to add extra managers to the childclass, but still use the default from AbstractBase
. You can’t add the newmanager directly in the child class, as that would override the default and you wouldhave to also explicitly include all the managers from the abstract base class.The solution is to put the extra managers in another base class and introduceit into the inheritance hierarchy after the defaults:
Note that while you can define a custom manager on the abstract model, youcan’t invoke any methods using the abstract model. That is:
is legal, but:
will raise an exception. This is because managers are intended to encapsulatelogic for managing collections of objects. Since you can’t have a collection ofabstract objects, it doesn’t make sense to be managing them. If you havefunctionality that applies to the abstract model, you should put that functionalityin a staticmethod
or classmethod
on the abstract model.
Implementation concerns¶
Whatever features you add to your custom Manager
, it must bepossible to make a shallow copy of a Manager
instance; i.e., thefollowing code must work:
Django makes shallow copies of manager objects during certain queries;if your Manager cannot be copied, those queries will fail.
This won’t be an issue for most custom managers. If you are justadding simple methods to your Manager
, it is unlikely that youwill inadvertently make instances of your Manager
uncopyable.However, if you’re overriding __getattr__
or some other privatemethod of your Manager
object that controls object state, youshould ensure that you don’t affect the ability of your Manager
tobe copied.
Description
Running your WordPress site with an e-Commerce plugin or solution? WP Affiliate Manager can help you manage an affiliate marketing program to drive more traffic and more sales to your store.
Affiliate Marketing is the fastest growing advertising method and it is very cost effective.
This plugin facilitates the affiliates recruitment, registration, login, management process.
It will also track the referrals your affiliates send to your site and give commissions appropriately.
Affiliates Manager integrates with some popular e-commerce solutions. It integrates with:
- WooCommerce
- Simple Shopping Cart
- WP eCommerce
- JigoShop
- Easy Digital Downloads
- iThemes Exchange
- WP eStore
- Sell Digital Downloads
- Paid Membership Pro
- S2Member
- Simple Membership
- Stripe Payments
Real-Time Reporting
Your affiliate’s traffic and sales are recorded and ready for display as soon as they happen.
Unlimited Affiliates
No matter if you have 1 or 1,000 affiliates, you can track them all.
Flat Rate or Percentage Based Payouts
You decide how you want to reward your affiliates. You can choose to pay your affiliates a flat rate per order, or choose to pay them a percentage of every order they initiate.
Set Payout Rates Per Affiliate
Each of your affiliates can be set to their own payout amount.
Url Manager Microsoft
Manual Adjustments and Payouts
There may be a time when you need to credit an affiliate for something other than a sale. For example, a bonus for reaching a sales goal. Manual adjustments are treated as a line item for easy tracking.
Pay Your Affiliates Using PayPal
Ability to easily pay your affiliates their commission using PayPal.
Unlimited Creatives and Ads for your Affiliates
You can add as many banners or text link ads as you wish. Plus, you can easily activate or deactivate creatives as needed.
Customizable Affiliate Registration
You can decide how much or how little data to collect from your affiliates when they sign up. You can mark each field as optional or required.
Autoresponder Integration
- Sign up affiliates to your Mailchimp list.
- Sign up affiliates to MailPoet newsletter list.
Affiliate Ad Impression Tracking
Track how many times a particular affiliate ad is getting viewed.
Customize Messages for Affiliates
You can customize the email messages that gets sent to your affiliate when they register for an account. The following messages are customizable:
- HTML message displayed to user at logon if affiliate STATUS = APPROVED
- HTML message displayed to user at logon if affiliate STATUS = DECLINED
- HTML message displayed to user at logon if affiliate STATUS = PENDING
- HTML message displayed to user after successfully submitting the affiliate registration form
- Body of the e-mail sent to the affiliate immediately after submitting their application.
- Body of the e-mail sent to a newly registered affiliate immediately following their application being approved.
- Body of e-mail sent to the affiliate immediately following their application being declined.
Translation Ready
This plugin can be translated to your language. The following language translations are already available in the plugin:
- English
- German
- French
- Hebrew
- Portuguese
- Spanish
- Bulgarian
- Finnish
- Dutch
- Italian
- Icelandic
- Polish
You can translate the plugin using this documentation.
Support
View the Plugin Documentation to get started.
If you have a question, you can ask it on our support forum.
Visit the affiliate manager plugin site for more details.
Developers
- If you are a developer and you need some extra hooks or filters for this plugin then let us know.
- Github repository – https://github.com/wp-insider/affiliates-manager-plugin
Url Manager Pro 5 1 – Bookmark Manager Resume
Arbitrary section
Url Manager Pro 5 1 – Bookmark Manager Software
None
Installation
- Go to the Add New plugins screen in your WordPress admin area
- Click the upload tab
- Browse for the plugin file (wpaffiliatemanager.zip)
- Click Install Now and then activate the plugin
Reviews
Mac Bookmark Manager
